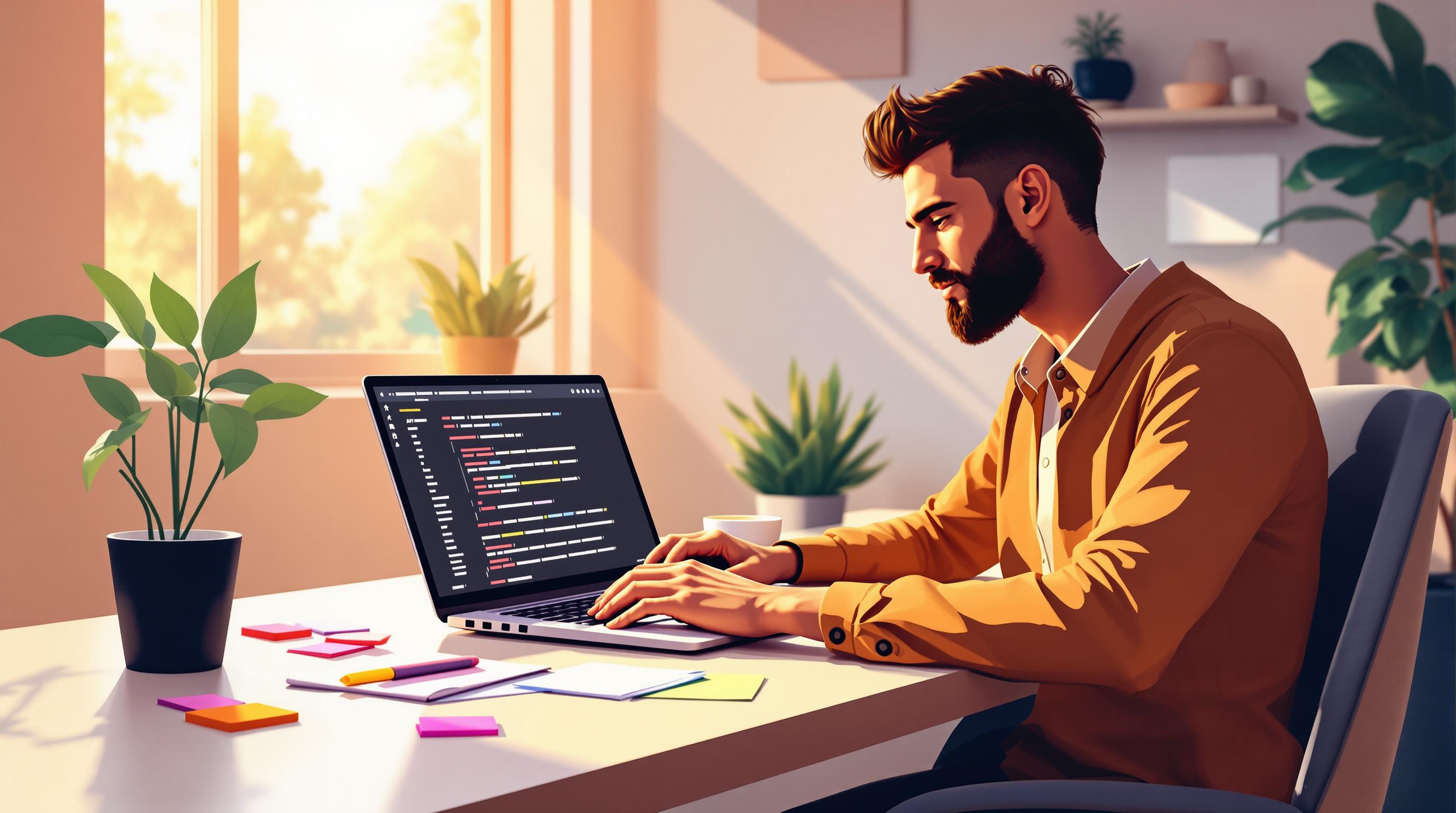
Learn how to create trustworthy API usage examples that address real developer needs through clarity, context, and error handling.
Want developers to trust your API? Start with clear, practical examples. Here's how you can create API documentation that developers actually rely on:
- Solve Real Problems: Show how your API fits into everyday developer workflows.
- Test and Update Regularly: Ensure examples are accurate and reflect the latest API version.
- Provide Context: Explain when, why, and how to use each example.
- Handle Errors: Include examples for common mistakes and edge cases.
- Use Popular Languages: Offer examples in languages your audience uses most.
Quick Example: A Python snippet for API authentication
import api_client
client = api_client.Client('YOUR_API_KEY')
response = client.get_data(parameter='value')
print(response.status_code)
Why it works: It's simple, functional, and ready to use. Developers can copy, paste, and adapt it immediately.
To maintain quality, test examples regularly, update them as APIs evolve, and collect feedback from users. By focusing on clarity and usability, you’ll create documentation that developers trust and use.
What Developers Need in Documentation
API documentation should be clear and thorough. Avoiding common pitfalls and including key elements can help establish trust with developers.
Common Documentation Problems
Developers often encounter these documentation issues:
- Outdated examples: Code samples that don’t match the current API version or rely on deprecated methods.
- Missing prerequisites: Lack of clear setup steps or information on required dependencies.
- Incomplete error scenarios: Examples that only show ideal outcomes while ignoring potential failures.
- Inconsistent formatting: Code snippets with syntax errors or that don’t follow standard conventions.
- Insufficient context: Examples that fail to explain their purpose or expected results.
Filling these gaps with clear, relevant examples ensures your API documentation is dependable.
Elements of Good Examples
Effective examples should include these key elements:
Element | Description |
---|---|
Completeness | Provide fully functional examples, including imports and setup instructions. |
Context | Explain how and when to use the examples in real projects. |
Consistency | Use standardized formatting and naming conventions throughout. |
Up-to-Date | Ensure examples reflect the latest API version. |
Error Handling | Show common errors and how to resolve them. |
Best Practices for Examples
Here are a few ways to apply these elements:
- Authentication and Setup: Offer detailed instructions for authentication and setup to simplify onboarding.
- Practical Context: Use examples that mirror real-world use cases, not overly simplified scenarios. Show how developers can integrate the API into their projects.
- Step-by-Step Complexity: Begin with basic examples and gradually introduce more complex implementations.
"The difference between good and great API documentation lies in its ability to anticipate and address developer needs before they become roadblocks."
- daily.dev Documentation Team
Building Clear API Examples
Creating clear and effective API examples means understanding your audience's technical needs and ensuring your examples address them directly.
Selecting Programming Languages
Choosing the right programming languages for your API examples can make your documentation more accessible and relevant to a wider audience. Including examples in multiple popular languages ensures they resonate with a diverse developer base.
Consideration | Action | Impact |
---|---|---|
Usage Statistics | Review GitHub language stats and Stack Overflow surveys | Focus on the most-used languages in your community |
Developer Profiles | Analyze the technical background of your user base | Align examples with your audience's expertise |
Implementation Complexity | Identify language-specific API challenges | Add extra details for languages with more complex integrations |
Once you've selected the languages, break down the implementation into easy-to-follow steps.
Writing Step-by-Step Instructions
Clear, sequential instructions build trust and make it easier for developers to follow along. Here's how to structure your examples:
-
Start with Prerequisites
Clearly outline what developers need before they begin:
- API authentication details
- Required dependencies and their versions
- Instructions for setting up the environment
-
Structure the Implementation
Organize the process into logical steps:
- Initialize the API client
- Configure basic settings
- Make the first API call
- Handle responses and potential errors
-
Provide Working Code
Include complete, ready-to-run code snippets. These should be tested and functional, so developers can use them immediately. For example:
# Example demonstrating authentication and basic API usage import api_client # Initialize the client with your API key client = api_client.Client('YOUR_API_KEY') # Set timeout and retry configurations client.configure(timeout=30, retries=3) # Make an API call try: response = client.get_data(parameter='value') print(f"Success: {response.status_code}") except api_client.APIError as e: print(f"Error: {e.message}")
Breaking down the process like this makes it easier for developers to understand and implement.
Including Helpful Context
Adding context to your examples helps developers grasp not just how to use your API, but also when and why. For each example, include:
- Use Case Description: Explain the real-world scenario the example addresses.
- Expected Outputs: Clearly state what results developers can expect and any performance considerations.
- Common Pitfalls: Point out potential issues and how to avoid them.
- Performance Considerations: Highlight any effects on rate limits or resource usage.
This extra context ensures developers not only implement your API correctly but also use it effectively in the right situations.
Writing Practical Examples
Provide clear, real-world API examples to demonstrate how your API solves common development challenges.
Common Use Cases
Start with examples that cover basic functionality, then move on to more complex scenarios.
Basic Operations Example:
# Retrieve user profile data
try:
user = api.users.get(user_id='12345')
# Access common profile fields
username = user.username
email = user.email
created_at = user.created_at
print(f"User {username} found")
except ApiError as e:
print(f"Failed to retrieve user: {e.message}")
Advanced Implementation Example:
# Batch process multiple users with rate limiting
from time import sleep
def process_users(user_ids, batch_size=100):
for i in range(0, len(user_ids), batch_size):
batch = user_ids[i:i + batch_size]
try:
results = api.users.bulk_get(user_ids=batch)
process_results(results)
# Handle rate limits
sleep(1)
except RateLimitError:
# Wait for rate limit reset
sleep(60)
continue
These examples not only show basic and advanced operations but also emphasize the importance of error handling.
Error Handling Examples
Error handling is essential for robust API usage. Include examples that address both routine errors and unexpected issues.
import requests
from requests.exceptions import RequestException
from time import sleep
def make_api_call(retry_count=3):
for attempt in range(retry_count):
try:
response = api.endpoint.call()
return response
except ConnectionError as e:
if attempt == retry_count - 1:
raise
sleep(2 ** attempt) # Exponential backoff
except ApiError as e:
if e.status_code >= 500:
# Retry server errors
continue
raise # Re-raise client errors
This approach ensures your API can handle errors gracefully, even under challenging conditions.
Multi-Endpoint Examples
Complex workflows often involve multiple API endpoints. Use examples to clarify these workflows step by step.
def register_new_user(email, username):
# 1. Create user account
user = api.users.create(
email=email,
username=username
)
# 2. Set up default preferences
preferences = api.preferences.create(
user_id=user.id,
defaults={'theme': 'light', 'notifications': True}
)
# 3. Generate API key for the user
api_key = api.keys.generate(
user_id=user.id,
scope=['read', 'write']
)
return {
'user': user,
'preferences': preferences,
'api_key': api_key
}
When building multi-endpoint examples, make sure to:
- Clearly outline the sequence of operations
- Address error handling at each step
- Include rollback logic for transactions, if needed
- Account for rate limits
- Highlight dependencies between calls
These examples help developers understand and implement complex workflows with confidence.
sbb-itb-e54ba74
Making Examples Easy to Use
Crafting practical examples is just the start. To make them truly helpful, focus on clear formatting and annotations that guide users toward quick understanding and easy implementation.
Code Formatting
Well-structured code examples are easier to read and apply. Follow consistent formatting and use syntax highlighting to improve clarity.
# Example of good formatting
def process_user_data(user_id: str) -> dict:
try:
# Fetch user profile
user = api.users.get(user_id)
# Process user preferences
preferences = api.preferences.get(
user_id=user_id,
include_defaults=True
)
return {
"user": user,
"preferences": preferences
}
except ApiError as e:
logger.error(f"Failed to process user {user_id}: {e}")
raise
Key principles for formatting:
- Use consistent indentation (4 spaces).
- Add line breaks to separate logical sections.
- Apply syntax highlighting to improve readability.
- Include type hints for better understanding.
- Break long lines to fit within a reasonable width.
This approach ensures your examples are not only visually clean but also ready for use.
Copy-Ready Code
Provide examples that are functional and can be executed with minimal changes. This saves users time and reduces potential errors.
# Fully functional, ready-to-use example
import requests
from typing import Optional
class ApiClient:
def __init__(self, api_key: str, base_url: str):
self.api_key = api_key
self.base_url = base_url.rstrip('/')
self.session = requests.Session()
self.session.headers.update({
'Authorization': f'Bearer {api_key}',
'Content-Type': 'application/json'
})
def get_user(self, user_id: str) -> Optional[dict]:
response = self.session.get(
f'{self.base_url}/users/{user_id}'
)
response.raise_for_status()
return response.json()
# Usage example
client = ApiClient(
api_key='your_api_key',
base_url='https://api.example.com/v1'
)
user = client.get_user('12345')
By offering complete, working code, you make it easier for developers to integrate your examples into their projects.
Code Comments
Strategic comments play a crucial role in explaining the purpose and logic behind your code. They help users understand complex parts without guessing.
# Example of effective commenting
def create_subscription(user_id: str, plan_id: str) -> dict:
# Check if the user is eligible for the subscription
eligibility = check_user_eligibility(user_id, plan_id)
if not eligibility.is_eligible:
raise ValidationError(eligibility.reason)
# Create a subscription with prorated billing
subscription = api.subscriptions.create(
user_id=user_id,
plan_id=plan_id,
start_date=get_billing_cycle_start(),
prorate=True
)
# Provision premium features immediately for premium plans
if subscription.plan.tier == 'premium':
provision_premium_features(user_id)
return subscription
Guidelines for comments:
- Clarify complex logic or business rules.
- Highlight assumptions and potential edge cases.
- Mention rate limits or timing considerations.
- Specify API version requirements if relevant.
Well-commented examples build trust and make your code easier to adapt to different workflows. By combining clear formatting, functional code, and thoughtful annotations, your examples become a reliable resource for developers.
Maintaining Example Quality
To keep API examples reliable and helpful, they must be regularly maintained. This involves testing, making updates, and incorporating developer feedback to ensure they remain relevant and effective.
Testing Examples
Automated testing is crucial for maintaining the quality of API examples. Here's a sample test suite:
# Example test suite for API examples
import pytest
from example_validator import ExampleValidator
def test_user_creation_example():
validator = ExampleValidator()
# Validate example with varied inputs
result = validator.validate_example(
'user_creation',
inputs={
'username': 'test_user',
'email': 'test@example.com'
},
expected_status=201
)
assert result.is_valid
assert result.response_matches_schema
Key testing practices include:
- Running examples through CI/CD pipelines with every API update
- Testing across multiple API versions
- Verifying examples against the latest API specifications
- Ensuring response formats align with current standards
Once testing is in place, managing updates ensures examples continue to meet developer needs.
Keeping Examples Current
A structured approach is essential for keeping examples up-to-date. Monitor API changes and revise examples promptly to avoid confusion.
Here’s an example of a version compatibility matrix:
API Version | Example Status | Verified | Required Updates |
---|---|---|---|
v2.1.0 | Current | Mar 15, 2025 | None needed |
v2.0.0 | Deprecated | Mar 1, 2025 | Update auth flow |
v1.9.0 | Legacy | Feb 1, 2025 | Mark as obsolete |
Guidelines for updates:
- Keep changelogs and code comments accurate
- Clearly label deprecated examples or remove them
- Update examples to reflect current best practices
Getting Developer Input
Developer feedback is the final piece in maintaining high-quality examples. By gathering input from developers, you can ensure examples remain practical and solve real-world problems.
Here’s an example of a feedback collection endpoint:
# Example feedback collection endpoint
@app.route('/feedback/example/<example_id>', methods=['POST'])
def collect_example_feedback(example_id):
feedback = request.json
# Record feedback
db.example_feedback.insert({
'example_id': example_id,
'rating': feedback['rating'],
'issues': feedback['issues'],
'suggestions': feedback['suggestions'],
'context': {
'language': feedback['language'],
'framework': feedback['framework'],
'use_case': feedback['use_case']
}
})
return {'status': 'success'}
Ways to gather feedback:
- Add feedback buttons directly in documentation
- Use GitHub issue templates for suggestions or improvements
- Conduct developer surveys
- Track usage analytics
- Hold interviews with experienced users
Monitor feedback metrics to identify improvement areas:
Metric | Target | Current (Mar 2025) |
---|---|---|
Example Success Rate | >95% | 93.2% |
Copy/Paste Usage | >80% | 85.7% |
Documentation Time Spent | <2 min | 1.8 min |
Support Tickets Related to Examples | <5% | 4.3% |
Reach Developers with daily.dev Ads
Promote your API documentation to the right audience. With daily.dev Ads, you can connect directly with developers who need your API resources.
Developer-Focused Targeting
daily.dev Ads allows you to focus on developers based on their specific technical profiles:
Targeting Criteria | How It Helps Your API Documentation |
---|---|
Programming Languages | Connect with developers using languages supported by your API. |
Seniority Levels | Reach experienced developers who can leverage advanced API features. |
Tool Usage | Engage developers working with tools and frameworks that complement your API. |
Global Reach | Expand your audience by targeting developers worldwide. |
These targeting options align smoothly with daily.dev Ads' ad placement formats.
Ad Placement Options
-
In-Feed Native Ads
These ads appear naturally within the feed, showcasing new API features or usage examples. -
Post Page Ads
Positioned within technical discussions, these ads highlight detailed API guides and implementation tips when developers are actively problem-solving. -
Personalized Digest Ads (Coming Soon)
Tailored to individual developer interests, these ads deliver your API documentation directly to the right audience.
Track the Impact of Your Documentation
Use real-time metrics to understand how your API documentation is performing:
Metric | What It Tracks |
---|---|
Website Traffic | Number of visits to your API documentation pages. |
Resource Downloads | How often example code or related resources are downloaded. |
With 40% of developers on daily.dev identified as power users, your documentation reaches those eager to explore new technologies. The platform connects with 1 in 50 developers globally.
Setting Up Your Campaign
Design your campaign to maximize impact with these options:
Campaign Type | Minimum Investment | Ideal For |
---|---|---|
Self-Serve | $5,000 | Broad reach to over 1 million developers. |
Direct Campaign | $5,000 | Precise targeting based on specific technologies. |
With over 1 billion interactions, daily.dev provides massive exposure to help drive API adoption and integration success.
Conclusion
Your API documentation has the power to earn and maintain developer trust when it prioritizes clarity, usability, and consistency. By focusing on these areas, you can create a resource that developers turn to time and again.
Some key practices include offering detailed error handling examples, ensuring code snippets are well-formatted and easy to use, and regularly testing your documentation for accuracy. As daily.dev for Business puts it, "Developer trust is everything".
Meeting real developer needs is what makes documentation stand out:
Documentation Element | Developer Benefit |
---|---|
Step-by-Step Instructions | Easy-to-follow implementation |
Error Handling Examples | Better preparation for real-world use |
Multi-Endpoint Integration | Complete architectural guidance |
Tested, Current Examples | Reliable and up-to-date reference |
The better your documentation supports developers, the more likely they are to adopt and promote your API. Keeping examples updated and incorporating feedback ensures your documentation remains a go-to resource.
Great API documentation isn't static. It requires regular updates and attention to detail, from error handling to example accuracy. By doing this, you create a dependable tool that not only supports developers but also encourages them to integrate and recommend your API.